In this really basic example I show how is simple to write a microservice in Node.js to get and set key value pairs on Redis and to deploy them using Docker containers.
To try this example on your PC you only need to install Docker Desktop and Node.js then follow the described steps.
Retrieve the Redis container image based on Alpine Linux.
docker pull redis:alpine
Create and run a container from the Redis image.
docker run -it -d --name redis-server redis:alpine
Inspect the default bridge network of Docker Desktop to get the IP assigned to the Redis container, in the IPv4Address property.
docker inspect bridge
Create a directory for the Node.js webservice project and init it through npm.
mkdir node-redis-example-1
cd node-redis-example-1
npm init -y
Always using npm we install as dependencies Express.js to create the webservices and the Redis.js client to interact with the server.
npm i express
npm i redis
We set the start script in the package.json file, we will use it later through a Dockerfile.
{
"name": "node-redis-example-1",
"version": "1.0.0",
"description": "",
"main": "index.js",
"scripts": {
"start": "node index.js"
},
"keywords": [],
"author": "",
"license": "ISC",
"dependencies": {
"express": "^4.17.1",
"redis": "^3.0.2"
}
}
Create the index.js file which will contain the following logic.
// Import packages.
const express = require('express')
const redis = require('redis')
const { promisify } = require('util')
// Create and configure a webserver.
const app = express()
app.use(express.json())
// Create and configure a Redis client.
const redisClient = redis.createClient('6379', process.env.REDIS_SERVER_IP)
redisClient.on('error', error => console.error(error))
const redisSet = promisify(redisClient.set).bind(redisClient)
const redisGet = promisify(redisClient.get).bind(redisClient)
// Create an endpoint to set a key value pair.
app.post('/setValue', async (req, res) => {
if (req.body.key && req.body.value) {
try {
await redisSet(req.body.key, req.body.value)
res.send()
} catch (e) {
res.json(e)
}
} else {
res.status(400).json({ error: 'Wrong input.' })
}
})
// Create an endpoint to get a key value pair.
app.get('/getValue/:key', async (req, res) => {
if (!req.params.key) {
return res.status(400).json({ error: 'Wrong input.' })
}
try {
const value = await redisGet(req.params.key)
res.json(value)
} catch (e) {
res.json(e)
}
})
// Start the webserver.
app.listen(3000, () => {
console.log('Server is up on port 3000')
})
Create a Dockerfile to build the container image of our microservice.
FROM node:12-alpine
WORKDIR /app
COPY ["package.json", "package-lock.json*", "./"]
RUN npm install
COPY . .
EXPOSE 3000
CMD [ "npm", "start" ]
If you’ve used Visual Studio Code at this point you will have these files.

Build the image using the Dockerfile.
docker build -t node-redis-example-1:1.0.0 .
Create and run the container of our microservice, setting the IP of the Redis server container and exposing the webservice.
docker run -it -d -p 3000:3000 -e "REDIS_SERVER_IP=172.17.0.2" --name node-redis-example-1 node-redis-example-1:1.0.0
Now in the Docker Desktop dashboard you can see your two containers running.
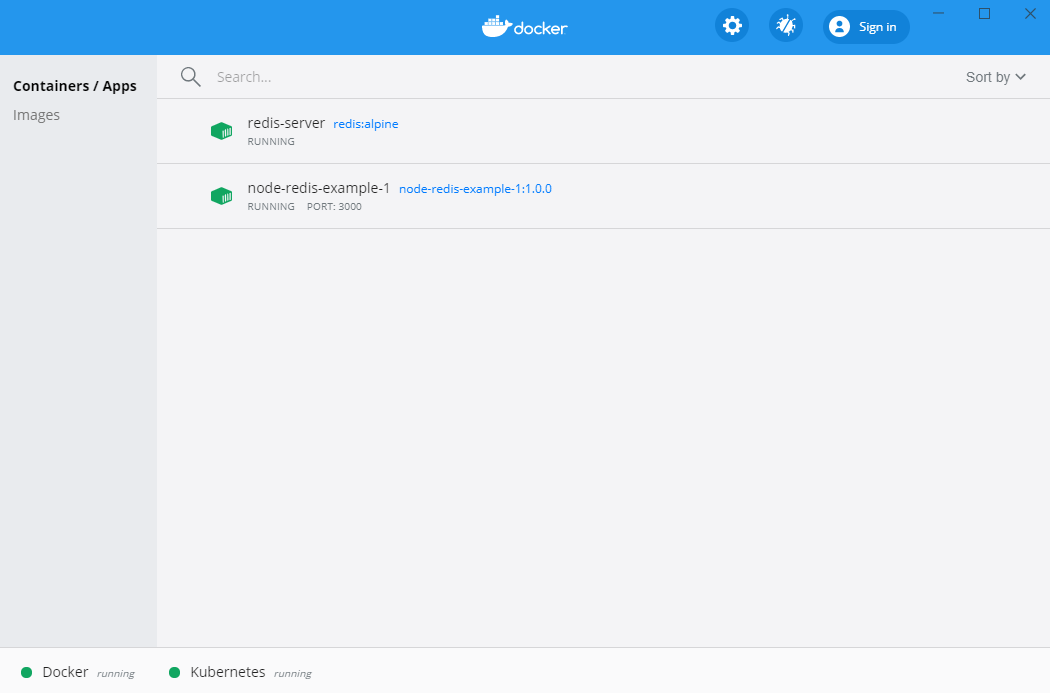
Just using Postman we can now try to set a key value pair.
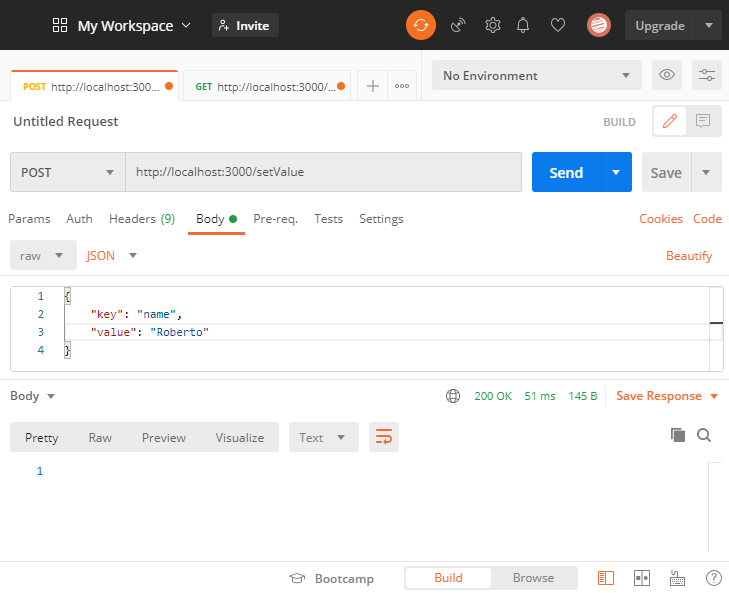
And then retrieve the saved value through its key.

You can find the source code on this GitHub repository:
https://github.com/robertobandini/node-redis-example-1
It also includes the Postman collection used and a sw-version.txt file to specify the softwares used for this project and their versions.
In the next posts I’ll talk about how to deploy it using Docker Compose and managing and resizing it via Kubernetes.